Python File Handling and Tricks By albro
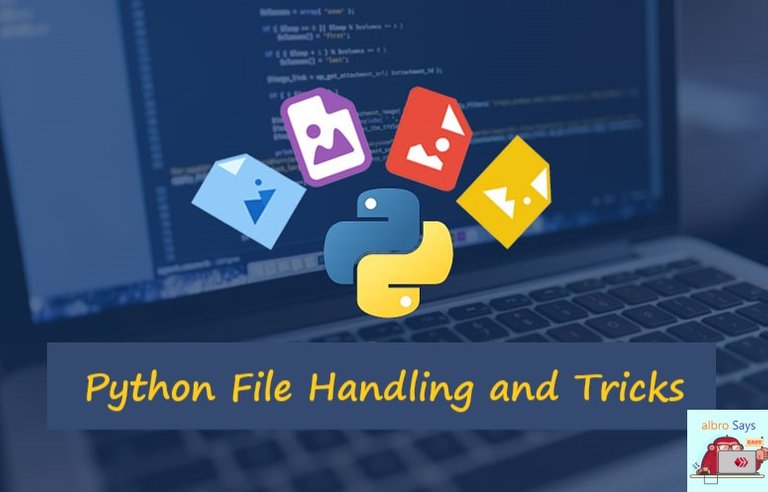
In programming with different purposes, we need to work with files. In this post, I'll talk about Python File Handling and its practical tricks.
To work with files in Python, we do not need to use and import any additional modules.
To work with files, we must use the File
object in Python. This object provides information about the file and simple functions so that we can work with files easily.
The first step to perform various operations on the file is to create an object from the desired File
. For this, we use the open()
function.
This function takes two input arguments; The first is the path and name of the file, and the second argument specifies the opening mode of the file.
file_name = "test.txt"
file = open( file_name, "a+" )
In this post, I use Python version 3; In version 2.7, the work process is similar and there may be small differences in the statement syntax.
Different file opening modes
As you know, there are different modes when opening a file for reading or writing. Some of the most important of them are:
r
: reading (starting from the beginning of the file)r+
: read and write (start from the beginning of the file)w
: create file to write (start from the beginning)w+
: create file for writing and reading (start from the beginning)a
: reading (starting from the end)a+
: reading and writing (starting from the end)
In the third and fourth cases (mode w
), if a file already exists, it deletes the file and creates a new file.
In two cases a
, if there is a file, it opens it and starts to perform operations from its end, and if there is no file, it creates an empty file.
There are other different modes for working with the file, the most used of which are the above.
Creating a file in Python
With these explanations, if you call the open()
function for a file that doesn't exist, that file will be created for you and you can put your data in it.
You can easily create a new file in any desired format and save your text data in it.
Note that when calling the open()
function, if the second argument is not specified and only the file name and path are given as input, the file will be created by default in r mode
and readable.
We store the value returned by the open()
function in a variable so that later we can call the functions we need on it and perform various operations on our file.
file_name = "test.txt"
file = open(file_name)
For better understanding, I use a help file that has the following contents:
dear @leo.voter, This is a test file! visit us at @albro blog on Hive blockchain Thanks @ecency, @xeldal, @minnowbooster and @chessbrotherspro
In the example codes, the commented items (using #
at the beginning of the line) mean the output of the code execution.
Different methods and functions can be used to read a text file in Python. In the following, we will examine three important functions and a practical trick together.
read
function
This function is called on the file object variable. Its output is a large text string containing all the contents of our file.
file_name = "test.txt" file = open(file_name) print( file.read() )
# dear @leo.voter, # This is a test file! # visit us at @albro blog on Hive blockchain # Thanks @ecency, @xeldal, @minnowbooster and @chessbrotherspro
Note that if we call this function twice in a row, the second time, no value will be printed for us! Because the entire file has been checked and read to the end and we have no more content to display.
The read()
function can have an optional input argument; This argument is of numeric type and specifies how many characters to read.
file = open(file_name)
print( file.read(7) )
# dear @l
readline
function to read file line by line in Python
This function returns one line of the file every time it is called on the file. That is, it can be called by the number of lines in the file (including empty lines).
file = open(file_name)
print( file.readline() )
print( file.readline() )
# dear @leo.voter,
#
# This is a test file!
#
Note that at the end of each line of the file, there is a newline character (\n
) and the print function that we use in the example also goes to a new line after each print
; That's why, as a result of the above code, we go to the new line twice for each line.
readlines
function
Sometimes, when reading a file in Python, we need to have all the contents of a file in a list. The first solution is to read the individual lines and put them into a Python list. But the readlines()
function does this for us very quickly.
By calling this function, we have a list as output that contains the lines in the file. That is, each member of this list will be equivalent to a line in the file.
file = open(file_name)
print( file.readlines() )
# ['dear @leo.voter,\n', 'This is a test file!\n', 'visit us at @albro blog on Hive blockchain\n', 'Thanks @ecency, @xeldal, @minnowbooster and @chessbrotherspro']
Use a loop to read the file
A simple way to read a file line by line in Python is to use Python's for loop or in other words for each
.
If we use the file variable in a loop, it is similar to calling the readlines()
function on it and giving the result list to the for
loop.
file = open(file_name)
for line in file:
print(line)
# dear @leo.voter,
#
# This is a test file!
#
# visit us at @albro blog on Hive blockchain
#
# Thanks @ecency, @xeldal, @minnowbooster and @chessbrotherspro
Close the file after working with the file in Python
Always make sure that when you are done with the open file, close it by calling the close()
function on the file object. Although the file is automatically closed after the Python program finishes executing, this will help free up resources sooner.
Also, if you are writing a file and the file isn't closed properly, nothing may be saved in the file. Somehow your changes won't be applied.
file.close()
Writing a file in Python
There are two main functions to write a text file in Python. In the following, we will examine these two functions together.
write
function to write the string in the file
This function takes a string as input and writes it into the file.
bot_settings = "settings.txt"
file = open(bot_settings, "w")
file.write("reblog = users.txt")
file.close()
By executing the above code, inside the settings.txt
file that existed or was created next to the program, only the value of reblog = users.txt
will be written
writelines
function
This function takes a list of text strings and writes them in order in the desired file.
file = open(bot_settings, "w")
file.writelines( ["reblog = users.txt\n", "upvote = voters.txt"] )
file.close()
Adding content to existing content in a Python file
In the section introducing the modes of opening a file, I said that if we open the existing file in w mode
, all the previous contents will be deleted and the file will be ready for new content to be written.
If we want to add new content to the current content of a file, we should open the file in a mode
(the beginning of the word append) and then write the file.
Delete file in Python
To delete a file in Python, we need a system module. This module or library exists by default in Python and we can add it to our program by adding a module called os
.
Therefore, at the beginning of our program, we write the following code.
import os
This module has a function called remove()
, which is called and given the file name as input, the desired file is deleted. Take a look at the code below for a better understanding. (Learn more: Modules in Python)
os.remove("settings.txt")
Here the settings.txt
file is next to the main Python file. If your file is located somewhere else, you can specify its path as input to this function.
To avoid errors when deleting a file, it is better to first check if the file exists in the desired path and then proceed to delete it.
For this, we can use other functions in the os
module and perform the deletion operation like the code below.
if os.path.exists("delete_file.txt"):
os.remove("delete_file.txt")
Error handling in Python file reading
When working with files in Python, we may encounter various errors. These errors have various reasons and it is better to refer to the text of the error message to fix it.
Common reasons for errors in writing or reading a Python file include:
- The desired file is being used by another program. (to be unlocked again by the locked operating system)
- The path of the wrong file was entered and there is no such path.
- The file name is not entered correctly and illegal characters are used in it.
Most of these errors occur during the execution of the program and cannot be predicted in advance. It is better to always use try except
blocks to work with files in Python.
A simple example of trying to open a file in Python is as follows.
try:
open("testFile.txt")
except:
print("An Error Occurred While Opening File!")
Summary: File in Python
In this work post I talked about file in Python language. In the beginning, I said that opening a file has different modes that we can use according to our needs.
I checked the file reading methods in Python. The open()
function is used to open the file. This function takes an input which is the address and name of the desired file. We learned about 3 main functions for working with files (for example, reading a txt file):
read
: To read the entire content of the filereadline
: To read the file line by linereadlines
: Convert file content to list
Then the methods of writing files in Python were explained. We have seen that it is very easy to work with files in Python to write information and we can write our desired content in the file with the help of write()
or writelines()
functions.
Finally, os.remove()
was introduced to delete the file. Also, in an example, I used the try except
structure to prevent errors from reading the file in Python.
https://leofinance.io/threads/view/albro/re-leothreads-rzpxsgkx
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 7000 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Check out our last posts:
Support the HiveBuzz project. Vote for our proposal!
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🏅 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.
Nice 👌
😍